Motors
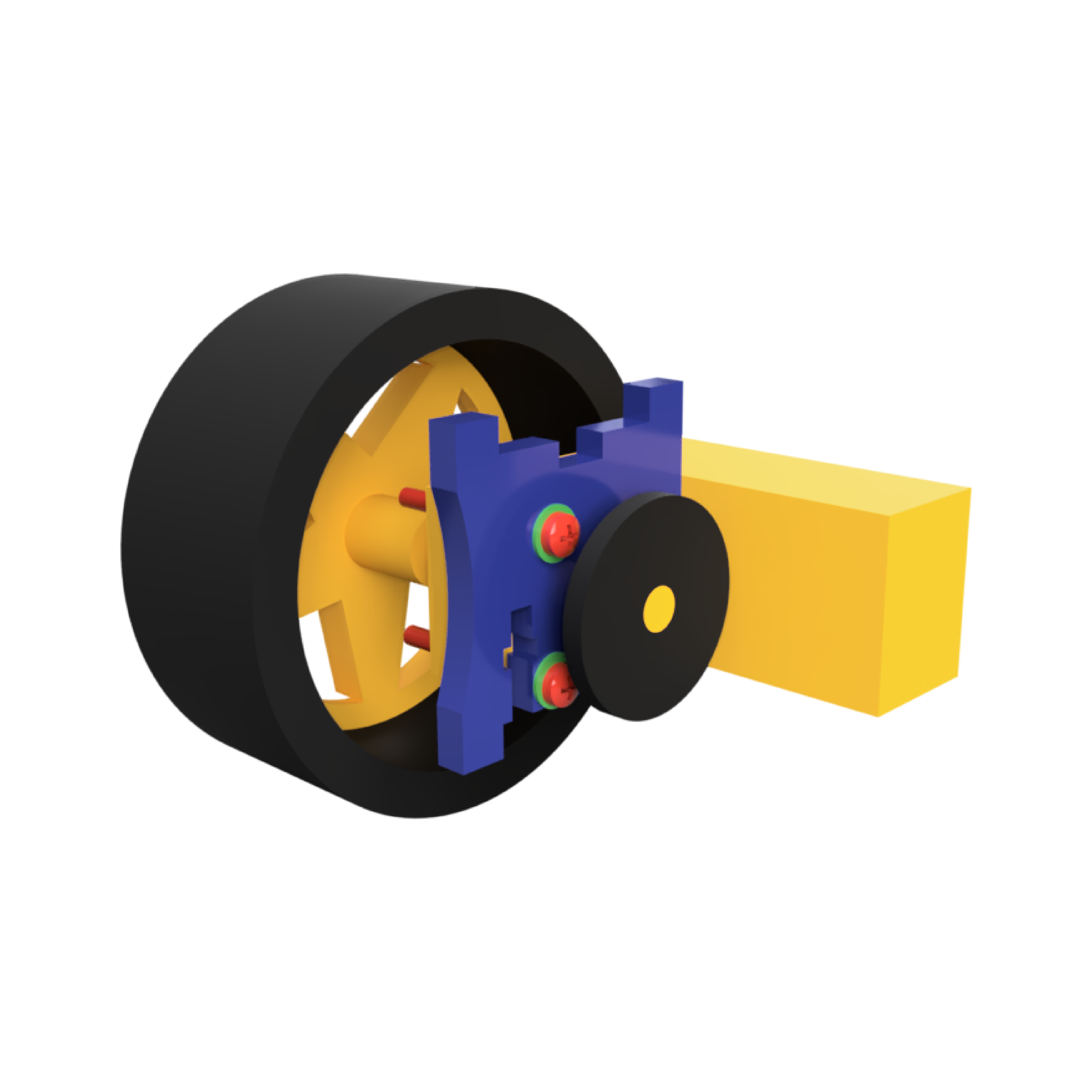
Now let's start with the motors that provide the propulsion for the Joy-Car. You can use the motors to move the Joy-Car both forwards and backwards. You can also adjust the speed individually, steer the vehicle in different directions and stop it if necessary. The motors therefore give you full control over the movements of your Joy-Car, allowing you to perform both precise maneuvers and dynamic driving.
You can find more information about the motors here.
The following code example shows the possible movements of the Joy-Car in detail. The Joy-Car can not only move in the basic directions forwards, backwards, to the right and to the left, but can also drive in a defined radius. This enables the Joy-Car to perform more complex maneuvers by making smooth turns and twists. The movement is controlled via specific commands that define the desired direction and, if necessary, the radius of the curve. This allows the Joy-Car to navigate flexibly and precisely through different environments.
Driving
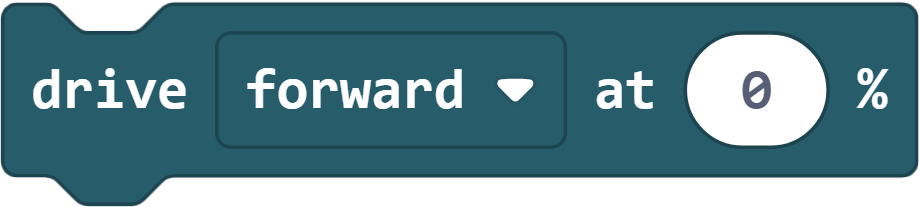
Drive forwards or backwards. You can also select the speed as a percentage between 0 (no drive) and 100 (maximum drive).
Braking
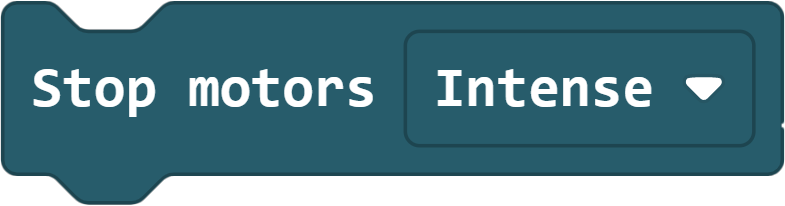
Brake the Joy-Car to a standstill. You can also choose between hard emergency braking or gentle braking, during which the Joy-Car slowly coasts to a stop.
Curves
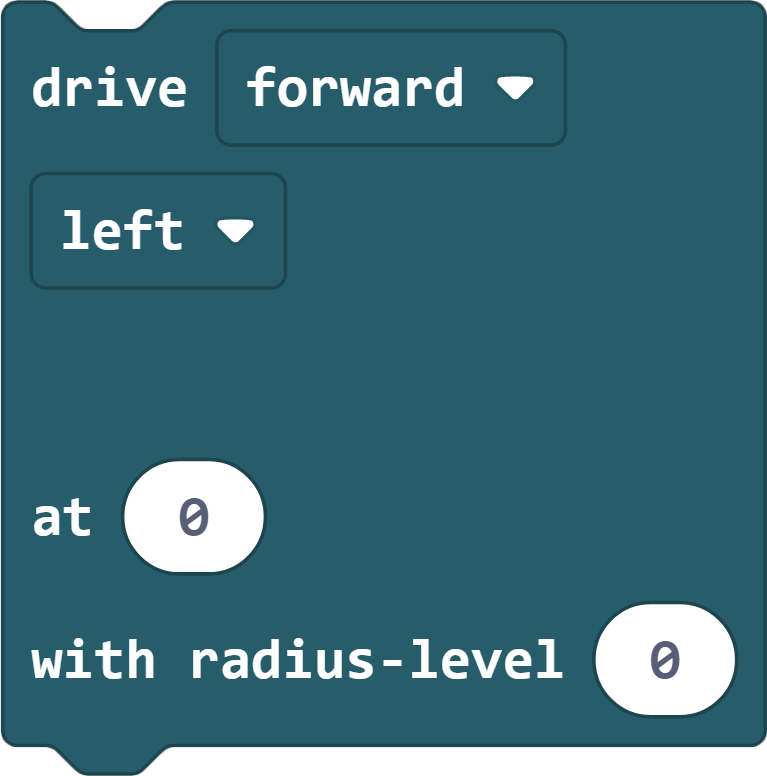
Drive a left or right turn. You can also set the speed as a percentage here. You can also specify the radius level of the curve from 0 - 5 (0 = tight curve, 5 = wide curve).
PWM signal
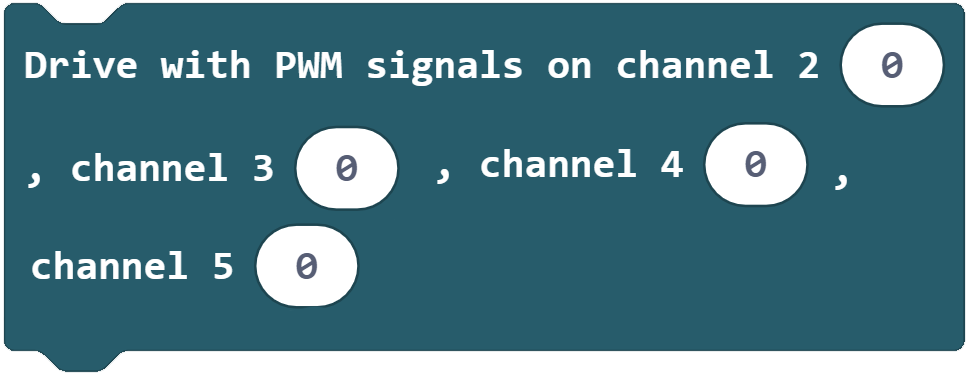
The motors can also be controlled directly via PWM signals. For this purpose, a PWM value between 0 and 255 can be sent to each channel.
Code example
Preparation for motors
First, we activate the motor control by sending the corresponding commands via the I2C interface.
This enables communication between the microcontroller and the motors. In order to control the motors precisely and efficiently, we use the variables biasL
and biasR
. These variables are used to adjust the rotational speeds of the left and right motors as a percentage. This is particularly important to compensate for deviations that can occur due to wear, manufacturing tolerances or other influences, and ensures that the Joy-Car drives in a straight line or can perform precise maneuvers.
Motor control
The drive method enables the two motors of the Joy-Car to be controlled. The PWM signals PWM0
and PWM1
control the left motor, while PWM2
and PWM3
are responsible for the right motor. Within the method, the input values are first scaled in order to adapt the motors to each other if there are differences in the rotational speeds. This scaling takes into account the previously defined correction factors such as biasL
and biasR
.
At the end of the method, the calculated motor speed is sent via the I2C interface to the motor controller, which uses the address 0x70
. This ensures that the control commands are implemented precisely and the motors operate at the desired speed.