Battery voltage
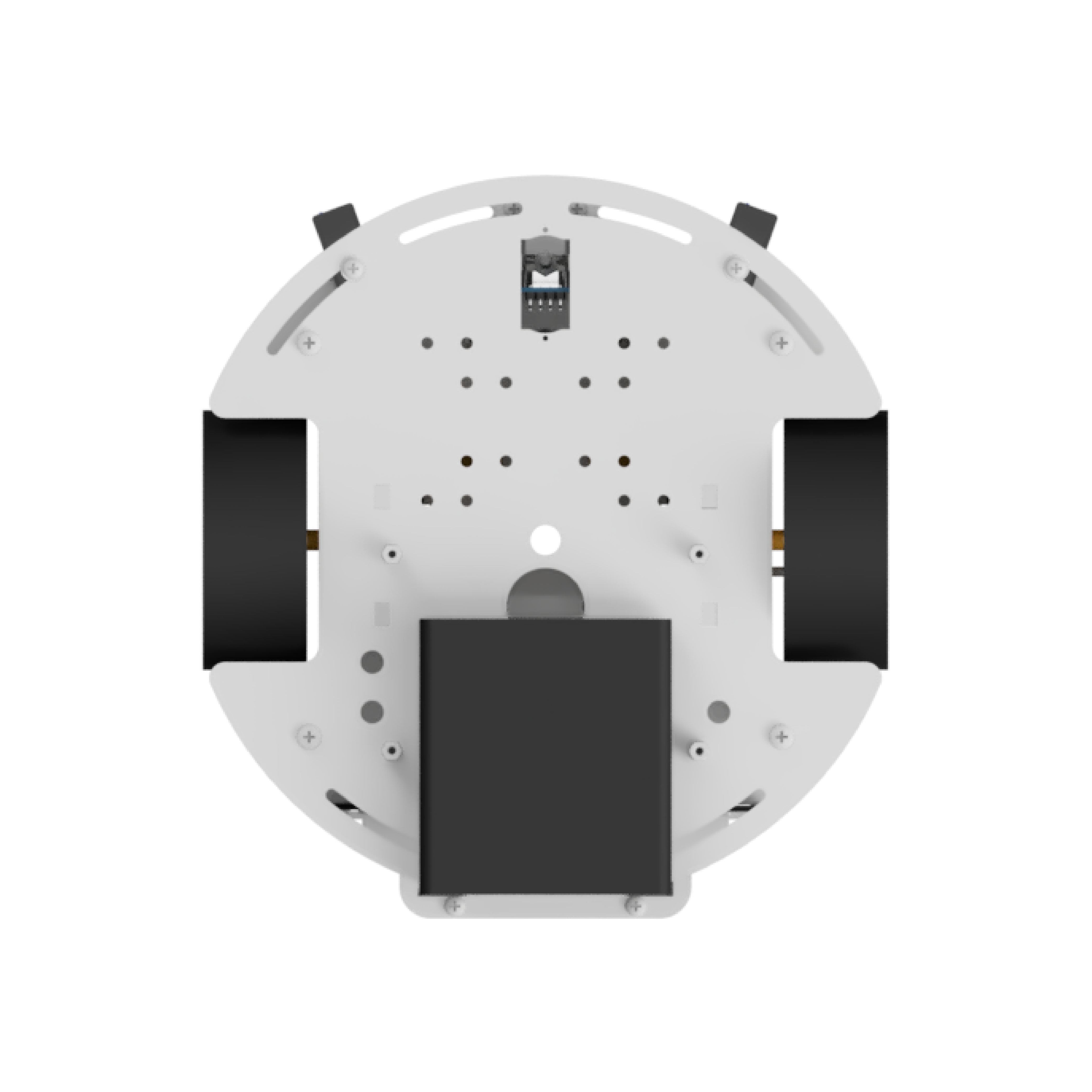
The Joy-Car has a battery compartment that ensures the vehicle's power supply. An analog pin is connected to this battery compartment, which is connected to an operational amplifier. The operational amplifier has a specific task here: it functions as a so-called impedance converter. In simple terms, this means that it transmits the voltage of the battery in such a way that it can be measured very accurately - without the measurement interfering with the battery or the circuit. This allows us to read and monitor the voltage of the battery compartment via the analog pin.
This function makes it possible to check the battery's state of charge and take action in good time if the voltage becomes too low to prevent the Joy-Car from failing.
In the following example, the voltage of the battery compartment, which is present at an analog pin of the Joy-Car, is read out. The analog voltage value recorded by the pin is then converted into volts. After the conversion, the voltage is output in volts in order to monitor the current charge status of the battery.
Battery voltage
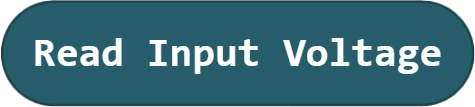
The battery voltage can be queried via the analog pin of the micro:bit. For example, the remaining battery capacity can be determined.
Code example
JoyCar.initJoyCar(RevisionMainboard.OnepThree)
basic.forever(function () {
serial.writeLine("Input voltage: " + JoyCar.readAdc() + " V")
basic.pause(1000)
})
Calculation
First, constants are defined that are required for calculating the battery voltage. These constants take into account the properties of the micro:bit's internal analog-to-digital converter (ADC), such as the maximum input voltage and the voltage range of the battery compartment. The constants include the reference voltage uref
, which specifies the maximum voltage that the ADC can process. Another important constant is the resolution of the ADC uratio
, which describes the maximum digital value that the ADC can output.
These constants are essential in order to correctly convert the digital values read out by the ADC into real voltage values in volts. They ensure that the battery voltage can be precisely calculated and monitored.
# Variables required for the conversion
# Max. Voltage at ADC pin / ADC resolution
uref = 3.3 / 1024
# (R1 + R2) / R2, Voltage division ratio (in kOhm)
uratio = (10 + 5.6) / 5.6
Calculate power supply
The voltage of the battery compartment is calculated in the supplyVoltage
method. First, the analog voltage value is read from pin 2 and stored in the variable adcvoltage
. This value represents the digital value measured by the ADC. The digital ADC value is then converted into a voltage value in the voltaged
variable using the previously defined constants, in particular the reference voltage (uref
). This corresponds to the voltage that was measured. Finally, the actual battery voltage is calculated in the voltagep
variable. The divider ratio of the voltage divider (uratio
) is taken into account in order to obtain the correct voltage of the battery compartment.
This method provides a precise calculation of the battery voltage, which can be used to monitor the state of charge.
# Method for calculating the supply voltage from the battery pack
def supplyVoltage():
# Read the ADC value
adcvoltage = pin2.read_analog()
# Convert value to voltage
voltaged = uref * adcvoltage
# Multiply the measured voltage by the voltage division ratio
# to calculate the actual voltage
voltagep = voltaged * uratio
return voltagep
Code example
# Import necessary libraries
from microbit import *
# Define your Joy-Car mainboard revision
joycar_rev = 1.3
# Variables required for the conversion
# Max. Voltage at ADC pin / ADC resolution
uref = 3.3 / 1024
# (R1 + R2) / R2, Voltage division ratio (in kOhm)
uratio = (10 + 5.6) / 5.6
# Method for calculating the supply voltage from the battery pack
def supplyVoltage():
# Read the ADC value
adcvoltage = pin2.read_analog()
# Convert value to voltage
voltaged = uref * adcvoltage
# Multiply the measured voltage by the voltage division ratio
# to calculate the actual voltage
voltagep = voltaged * uratio
return voltagep
# outputs the value from the supplyVoltage method
print("Input voltage = " + str(supplyVoltage()) + " V")